Обязательное условие: API данных Youtube для обработки видео | Набор-1, Набор-2
Прежде чем продолжить, сначала посмотрите, как получить список допустимых категорий видео, чтобы определить, в какую категорию поместить видео. Для примера мы использовали значение “В” для regionCode
параметра. Вы можете использовать любое другое значение. Только идентификаторы категорий, у которых assignable
параметр имеет значение “True”, могут использоваться при вставке или обновлении видео.
Давайте обсудим, как вставить видео в учетную запись авторизованного пользователя на YouTube.
Найдите допустимые категории видео:
# importing library
from apiclient.discovery import build
# Arguments that need to passed
# to the build function
DEVELOPER_KEY = "API_key"
YOUTUBE_API_SERVICE_NAME = "youtube"
YOUTUBE_API_VERSION = "v3"
# creating Youtube Resource Object
youtube_object = build(YOUTUBE_API_SERVICE_NAME,
YOUTUBE_API_VERSION,
developerKey = DEVELOPER_KEY)
def youtube_video_categories():
# calling the videoCategory.list method
# to retrieve youtube video categories result
video_category = youtube_object.videoCategories(
).list(part ='snippet', regionCode ='IN').execute()
# extracting the results
# from video_category response
results = video_category.get("items", [])
# empty list to store video category metadata
videos_categories = []
# extracting required info
# from each result object
for result in results:
# video_categories result object
videos_categories.append("% s, (% s % s)"
%(result["id"],
result["snippet"]["title"],
result["snippet"]["assignable"]))
print ("Video_Category_Id Catgeory_Name Assignable :\n",
"\n".join(videos_categories), "\n")
if __name__ == "__main__":
youtube_video_categories()
Выход:
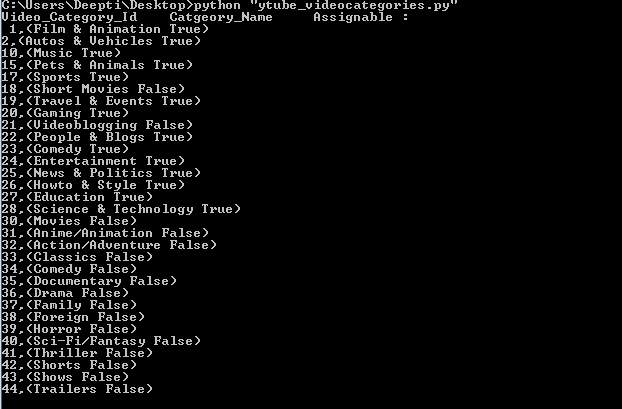
Поскольку для вставки видео в учетную запись авторизованного пользователя требуется авторизация пользователя, поэтому мы создадим учетные данные типа OAuth для этого примера. Выполните следующие действия, чтобы создать Идентификатор клиента и Секретный ключ.
- Перейдите в консоль разработчиков Google Google и нажмите «Войти» в правом верхнем углу страницы. Войдите в систему, используя учетные данные действительной учетной записи Google. Если у вас нет учетной записи Google, сначала настройте учетную запись, а затем используйте данные для входа на домашнюю страницу разработчиков Google.
- Теперь перейдите на панель инструментов разработчика и создайте новый проект.
- Нажмите на опцию Включить API.
- В поле поиска найдите API данных Youtube и выберите опцию API данных Youtube, которая находится в раскрывающемся списке.
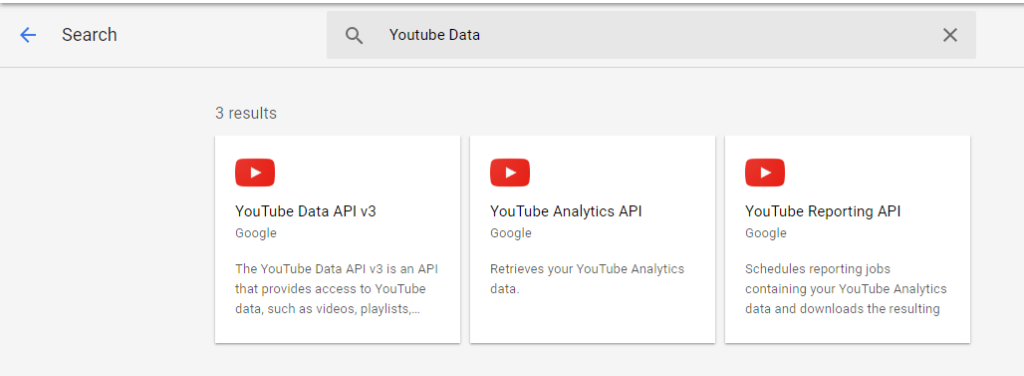
- Вы будете перенаправлены на экран с информацией об API данных Youtube, а также двумя вариантами : ВКЛЮЧИТЬ и ПОПРОБОВАТЬ API.
- Нажмите на опцию ВКЛЮЧИТЬ, чтобы начать работу с API.
- На боковой панели в разделе API и службы выберите Учетные данные.
- В верхней части страницы выберите вкладку Экран согласия OAuth. Выберите адрес электронной почты, введите название продукта, если оно еще не задано, и нажмите кнопку Сохранить.
- На вкладке Учетные данные выберите раскрывающийся список Создать учетные данные и выберите Идентификатор клиента OAuth. OAuth обычно используется там, где требуется авторизация, например, в случае получения понравившихся видео пользователя.
- Выберите тип приложения “Другое», введите имя «API данных YouTube Myvideos” и нажмите кнопку «Создать».
- Нажмите кнопку ОК.
- Нажмите на кнопку загрузки справа от идентификатора клиента, чтобы загрузить файл JSON.
- Сохраните и переименуйте файл как
client_secret.json
и переместите его в рабочий каталог.
Установите дополнительные библиотеки с помощью pip
команды:
pip install --upgrade google-auth google-auth-oauthlib google-auth-httplib2
Мы загрузили образец видео — Изучите азбуку, чтобы показать, как работает загрузка. Идентификатор, фрагмент .title и фрагмент .CategoryID являются обязательными свойствами, все остальные являются необязательными.
Вставка видео: В этом примере показано, как загрузить видео в учетную запись авторизованного пользователя. Загрузка видео регулируется следующими ограничениями:
- Файл может принимать максимальный размер 128 МБ
- Допустимыми типами MIME мультимедиа являются только видео/*, приложение/октетный поток
# importing necessary libraries
import os
import urllib.request, urllib.parse, urllib.error
import http.client
import urllib.request
import urllib.error
import http.client
import httplib2
import random
import time
import google.oauth2.credentials
import google_auth_oauthlib.flow
from googleapiclient.discovery import build
from googleapiclient.errors import HttpError
from google_auth_oauthlib.flow import InstalledAppFlow
from apiclient.http import MediaFileUpload
# The CLIENT_SECRETS_FILE variable
# specifies the name of a file that
# contains client_id and client_secret.
CLIENT_SECRETS_FILE = "client_secret.json"
# This scope allows for full read /
# write access to the authenticated
# user's account and requires requests
# to use an SSL connection.
SCOPES = ['https://www.googleapis.com/auth/youtube.force-ssl']
API_SERVICE_NAME = 'youtube'
API_VERSION = 'v3'
def get_authenticated_service():
flow = InstalledAppFlow.from_client_secrets_file(
CLIENT_SECRETS_FILE, SCOPES)
credentials = flow.run_console()
return build(API_SERVICE_NAME, API_VERSION,
credentials = credentials)
# Here we are telling HTTP Transport
# Library not to retry the video upload.
httplib2.RETRIES = 1
# MAX_RETRIES specifies the maximum number
# of retries that can done before giving up.
MAX_RETRIES = 10
# Always retry when these exceptions are raised.
RETRIABLE_EXCEPTIONS = (httplib2.HttpLib2Error, IOError,
http.client.NotConnected,
http.client.IncompleteRead,
http.client.ImproperConnectionState,
http.client.CannotSendRequest,
http.client.CannotSendHeader,
http.client.ResponseNotReady,
http.client.BadStatusLine)
# Always retry when an apiclient.errors.HttpError
# with one of these status codes is raised.
RETRIABLE_STATUS_CODES = [500, 502, 503, 504]
# This method implements an exponential
# backoff strategy to resume a failed upload.
def resumable_upload(request, resource, method):
response = None
error = None
retry = 0
while response is None:
try:
print("Uploading the file...")
status, response = request.next_chunk()
if response is not None:
if method == 'insert' and 'id' in response:
print(response)
elif method != 'insert' or 'id' not in response:
print(response)
else:
exit("The file upload failed with an\
unexpected response: % s" % response)
except HttpError as e:
if e.resp.status in RETRIABLE_STATUS_CODES:
error = "A retriable HTTP error % d occurred:\n % s"
% (e.resp.status, e.content)
else:
raise
except RETRIABLE_EXCEPTIONS as e:
error = "A retriable error occurred: % s" % e
if error is not None:
print(error)
retry += 1
if retry > MAX_RETRIES:
exit("No longer attempting to retry.")
max_sleep = 2 ** retry
sleep_seconds = random.random() * max_sleep
print(("Sleeping % f seconds and then retrying..."
% sleep_seconds))
time.sleep(sleep_seconds)
def print_response(response):
print(response)
# Build a resource based on a list of
# properties given as key-value pairs.
# Leave properties with empty values
# out of the inserted resource.
def build_resource(properties):
resource = {}
for p in properties:
# Given a key like "snippet.title", split into
# "snippet" and "title", where
# "snippet" will be an object and "title"
# will be a property in that object.
prop_array = p.split('.')
ref = resource
for pa in range(0, len(prop_array)):
is_array = False
key = prop_array[pa]
# For properties that have array values,
# convert a name like "snippet.tags[]" to
# snippet.tags, and set a flag to handle
# the value as an array.
if key[-2:] == '[]':
key = key[0:len(key)-2:]
is_array = True
if pa == (len(prop_array) - 1):
# Leave properties without values
# out of inserted resource.
if properties[p]:
if is_array:
ref[key] = properties[p].split(', ')
else:
ref[key] = properties[p]
elif key not in ref:
# For example, the property is "snippet.title",
# but the resource does not yet have a "snippet"
# object. Create the snippet object here.
# Setting "ref = ref[key]" means that in the
# next time through the "for pa in range ..." loop,
# we will be setting a property in the
# resource's "snippet" object.
ref[key] = {}
ref = ref[key]
else:
# For example, the property is "snippet.description",
# and the resource already has a "snippet" object.
ref = ref[key]
return resource
# Remove keyword arguments that are not set
def remove_empty_kwargs(**kwargs):
good_kwargs = {}
if kwargs is not None:
for key, value in list(kwargs.items()):
if value:
good_kwargs[key] = value
return good_kwargs
def videos_insert(client, properties, media_file, **kwargs):
resource = build_resource(properties)
kwargs = remove_empty_kwargs(**kwargs)
request = client.videos().insert(body = resource,
media_body = MediaFileUpload(media_file,
chunksize =-1, resumable = True), **kwargs)
return resumable_upload(request, 'video', 'insert')
if __name__ == '__main__':
# When running locally, disable OAuthlib's
# HTTPs verification. When running in production
# * do not * leave this option enabled.
os.environ['OAUTHLIB_INSECURE_TRANSPORT'] = '1'
client = get_authenticated_service()
media_file = 'videoplayback.3gp'
if not os.path.exists(media_file):
exit('Please specify the complete valid file location.')
videos_insert(client,
{'snippet.categoryId': '27',
'snippet.defaultLanguage': '',
'snippet.description': 'Sample Leran ABC Video',
'snippet.tags[]': '',
'snippet.title': 'Sample Test video upload',
'status.embeddable': '',
'status.license': '',
'status.privacyStatus': 'private',
'status.publicStatsViewable': ''},
media_file,
part ='snippet, status')
Выход:
При выполнении кода он запросит код авторизации. Для получения кода вам необходимо перейти по ссылке, указанной на экране командной строки над строкой: Введите код авторизации.

Теперь перейдите по ссылке и скопируйте код авторизации, который вы получите, предоставив разрешение.
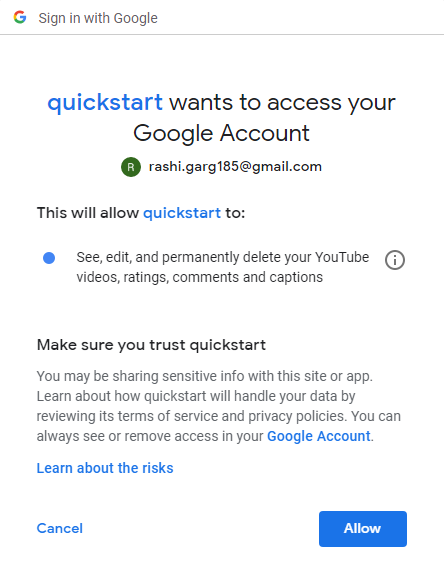
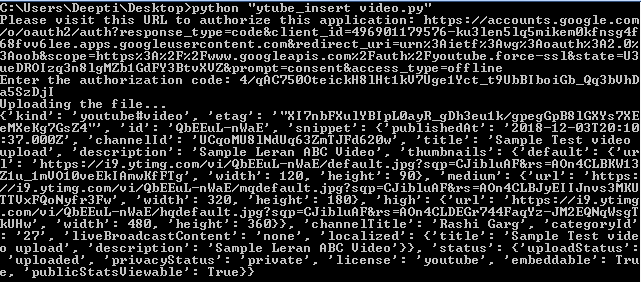
Теперь, как вы можете видеть из используемого аккаунта, он показывает, что видео загружено.
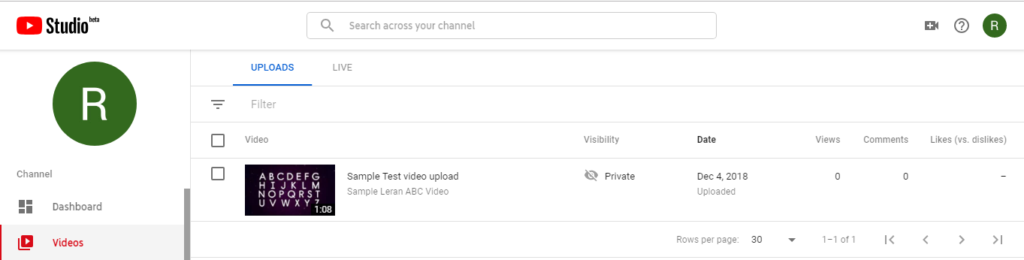
Рекомендации: