Предварительное условие: Глобальные и локальные переменные в JavaScript
Локальная область: Переменные, объявленные внутри функции, называются локальными переменными, и доступ к ним осуществляется только внутри функции. Локальные переменные удаляются после завершения функции.
Глобальная область действия: Доступ к глобальным переменным можно получить как внутри функции, так и за ее пределами. Они удаляются, когда окно браузера закрыто, но доступны для других страниц, загруженных в том же окне. Существует два способа объявить переменную глобально:
- Объявите переменную вне функций.
- Назначьте значение переменной внутри функции, не объявляя ее с помощью ключевого слова “var”.
<!DOCTYPE html>
<html>
<head>
<title>
How to change the value of a global
variable inside of a function using
JavaScript?
</title>
<script>
// Declare global variables
var globalFirstNum1 = 9;
var globalSecondNum1 = 8;
function add() {
// Access and change globalFirstNum1 and globalSecondNum1
globalFirstNum1 = Number(document.getElementById("fNum").value);
globalSecondNum1 = Number(document.getElementById("sNum").value);
// Add local variables
var result = globalFirstNum1 + globalSecondNum1;
var output = "Sum of 2 numbers is " + result;
// Display result
document.getElementById("result").innerHTML = output;
}
// Declare global variables
globalFirstNum2 = 8;
globalSecondNum2 = 9;
function subtract() {
// Access and change globalFirstNum2
// and globalSecondNum2
globalFirstNum2 = Number(document.getElementById("fNum").value);
globalSecondNum2 = Number(document.getElementById("sNum").value);
// Use global variables to subtract numbers
var result = globalFirstNum2-globalSecondNum2;
var output = "Difference of 2 numbers is " + result;
document.getElementById("result").innerHTML = output;
}
</script>
</head>
<body style="text-align:center;">
<h1 style="color:green">
GeeksForGeeks
</h1>
<b>Enter first number :- </b>
<input type="number" id="fNum">
<br><br>
<b>Enter second number :- </b>
<input type="number" id="sNum">
<br><br>
<button onclick="add()">Add</button>
<button onclick="subtract()">Subtract</button>
<p id="result" style = "color:green; font-weight:bold;">
</p>
</body>
</html>
Выход:
- Прежде чем нажать на кнопку:
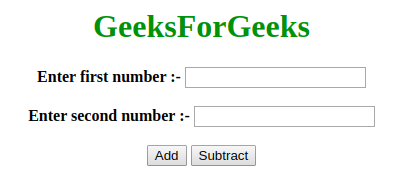
- После нажатия кнопки добавить:

- После нажатия кнопки вычесть:
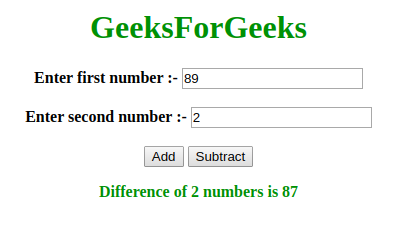