Необходимое условие: Декораторы на Python, Функциональные декораторы
Мы знаем, что декораторы — это очень мощный и полезный инструмент в Python, так как он позволяет программистам изменять поведение функции или класса. В этой статье мы узнаем о Декораторах с параметрами с помощью нескольких примеров.
Функции Python являются гражданами первого класса, что означает, что функции могут обрабатываться аналогично объектам.
- Функция может быть назначена переменной, т. е. на них можно ссылаться.
- Функция может быть передана в качестве аргумента другой функции.
- Функция может быть возвращена из функции.
Декораторы с параметрами аналогичны обычным декораторам.
Синтаксис для декораторов с параметрами:
@decorator(params)
def func_name():
''' Function implementation'''
Приведенный выше код эквивалентен
def func_name():
''' Function implementation'''
func_name = (decorator(params))(func_name)
"""
Когда выполнение начинается слева направо, вызывается декоратор(параметры), который возвращает объект функции fun_obj. Используя fun_obj, выполняется вызов fun_obj(имя fun_name). Внутри внутренней функции выполняются необходимые операции и возвращается фактическая ссылка на функцию, которая будет присвоена func_name. Теперь функцию func_name() можно использовать для вызова функции с примененным к ней декоратором.
Как реализован декоратор с параметрами
def decorators(*args, **kwargs):
def inner(func):
'''
do operations with func
'''
return func
return inner #this is the fun_obj mentioned in the above content
@decorators(params)
def func():
"""
function implementation
"""
Здесь параметры также могут быть пустыми.
Сначала понаблюдайте за этими :
# Python code to illustrate
# Decorators basic in Python
def decorator_fun(func):
print("Inside decorator")
def inner(*args, **kwargs):
print("Inside inner function")
print("Decorated the function")
# do operations with func
func()
return inner
@decorator_fun
def func_to():
print("Inside actual function")
func_to()
Другой способ:
# Python code to illustrate
# Decorators with parameters in Python
def decorator_fun(func):
print("Inside decorator")
def inner(*args, **kwargs):
print("Inside inner function")
print("Decorated the function")
func()
return inner
def func_to():
print("Inside actual function")
# another way of using decorators
decorator_fun(func_to)()
Выход:
Inside decorator
Inside inner function
Decorated the function
Inside actual function
Давайте перейдем к другому примеру:
Пример № 1:
# Python code to illustrate
# Decorators with parameters in Python
def decorator(*args, **kwargs):
print("Inside decorator")
def inner(func):
# code functionality here
print("Inside inner function")
print("I like", kwargs['like'])
func()
# returning inner function
return inner
@decorator(like = "geeksforgeeks")
def my_func():
print("Inside actual function")
Выход:
Inside decorator
Inside inner function
I like geeksforgeeks
Inside actual function
Пример № 2:
# Python code to illustrate
# Decorators with parameters in Python
def decorator_func(x, y):
def Inner(func):
def wrapper(*args, **kwargs):
print("I like Geeksforgeeks")
print("Summation of values - {}".format(x+y) )
func(*args, **kwargs)
return wrapper
return Inner
# Not using decorator
def my_fun(*args):
for ele in args:
print(ele)
# another way of using decorators
decorator_func(12, 15)(my_fun)('Geeks', 'for', 'Geeks')
Выход:
I like Geeksforgeeks
Summation of values - 27
Geeks
for
Geeks
Этот пример также говорит нам, что внешние параметры функции могут быть доступны с помощью заключенной внутренней функции.
Пример № 3:
# Python code to illustrate
# Decorators with parameters in Python (Multi-level Decorators)
def decodecorator(dataType, message1, message2):
def decorator(fun):
print(message1)
def wrapper(*args, **kwargs):
print(message2)
if all([type(arg) == dataType for arg in args]):
return fun(*args, **kwargs)
return "Invalid Input"
return wrapper
return decorator
@decodecorator(str, "Decorator for 'stringJoin'", "stringJoin started ...")
def stringJoin(*args):
st = ''
for i in args:
st += i
return st
@decodecorator(int, "Decorator for 'summation'\n", "summation started ...")
def summation(*args):
summ = 0
for arg in args:
summ += arg
return summ
print(stringJoin("I ", 'like ', "Geeks", 'for', "geeks"))
print()
print(summation(19, 2, 8, 533, 67, 981, 119))
Выход:
Decorator for 'stringJoin'
Decorator for 'summation'
stringJoin started ...
I like Geeksforgeeks
summation started ...
1729
1. Внутри декоратора
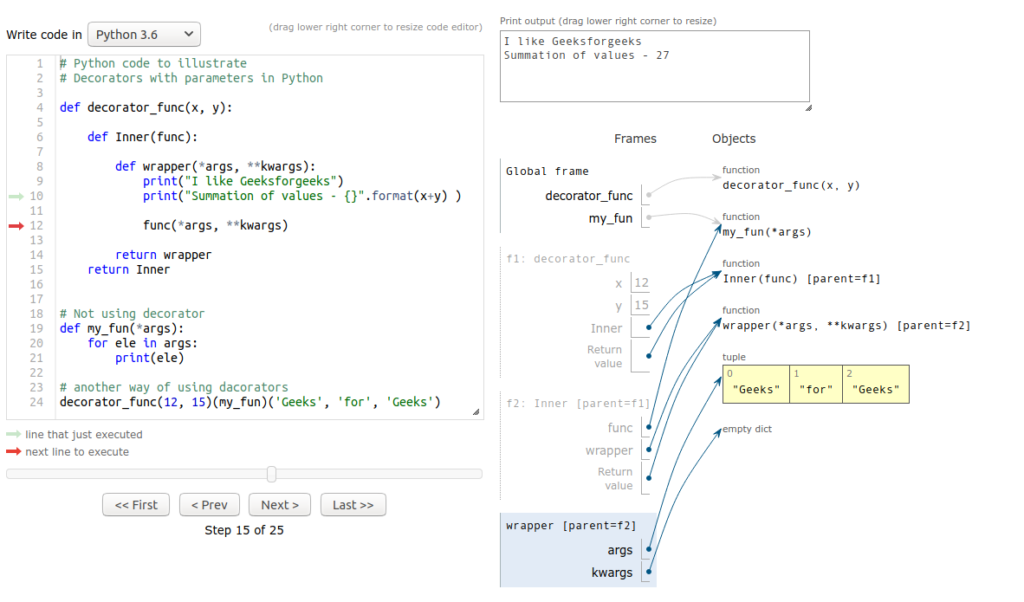
2. Внутри функции
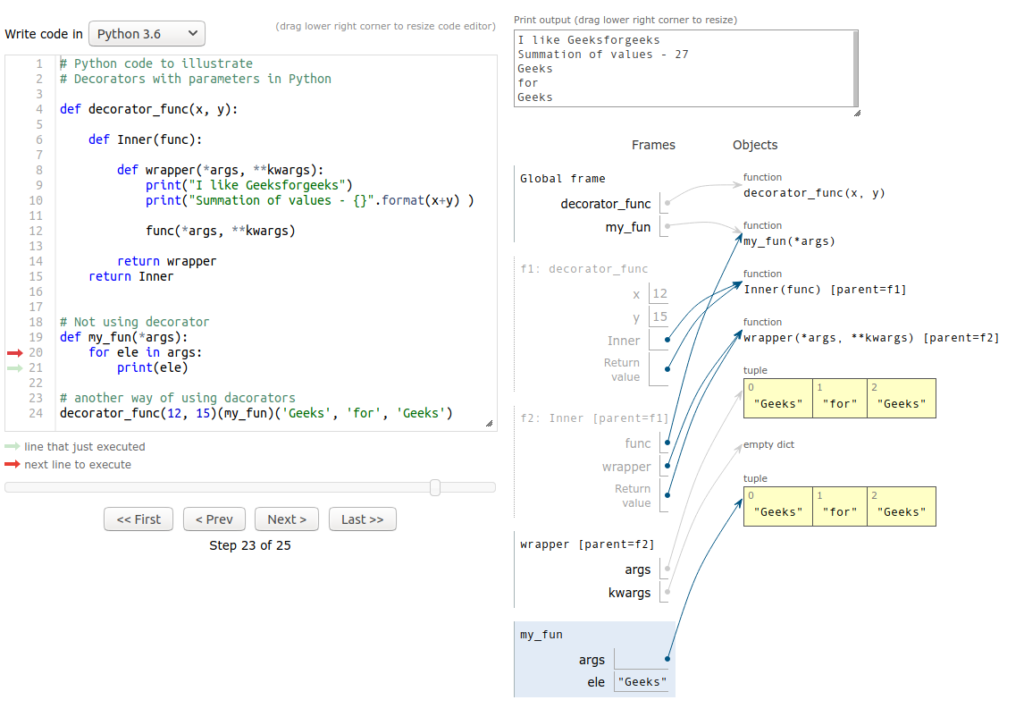
Примечание: Снимки изображений делаются с помощью PythonTutor.