Используя модуль xlrd, можно извлекать информацию из электронной таблицы. Например, чтение, запись или изменение данных можно выполнять на языке Python. Кроме того, пользователю, возможно, придется просматривать различные листы и извлекать данные на основе некоторых критериев или изменять некоторые строки и столбцы, а также выполнять большую работу.
модуль xlrd используется для извлечения данных из электронной таблицы.
Команда для установки модуля xlrd :
pip install xlrd
Входной файл:
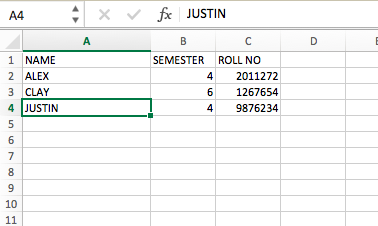
Код № 1: Извлеките определенную ячейку
# Reading an excel file using Python
import xlrd
# Give the location of the file
loc = ("path of file")
# To open Workbook
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index(0)
# For row 0 and column 0
print(sheet.cell_value(0, 0))
Выход:
'NAME'
Код № 2: Извлеките количество строк
# Program to extract number
# of rows using Python
import xlrd
# Give the location of the file
loc = ("path of file")
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index(0)
sheet.cell_value(0, 0)
# Extracting number of rows
print(sheet.nrows)
Выход:
4
Код № 3: Извлеките количество столбцов
# Program to extract number of
# columns in Python
import xlrd
loc = ("path of file")
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index(0)
# For row 0 and column 0
sheet.cell_value(0, 0)
# Extracting number of columns
print(sheet.ncols)
Выход:
3
Код № 4 : Извлечение имени всех столбцов
# Program extracting all columns
# name in Python
import xlrd
loc = ("path of file")
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index(0)
# For row 0 and column 0
sheet.cell_value(0, 0)
for i in range(sheet.ncols):
print(sheet.cell_value(0, i))
Выход:
NAME SEMESTER ROLL NO
Код № 5: Извлеките первую колонку
# Program extracting first column
import xlrd
loc = ("path of file")
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index(0)
sheet.cell_value(0, 0)
for i in range(sheet.nrows):
print(sheet.cell_value(i, 0))
Выход:
NAME ALEX CLAY JUSTIN
Код № 6: Извлеките определенное значение строки
# Program to extract a particular row value
import xlrd
loc = ("path of file")
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index(0)
sheet.cell_value(0, 0)
print(sheet.row_values(1))
Выход:
['ALEX', 4.0, 2011272.0]